[Kotlin] μ½νλ¦° Property μμ
μλ νμΈμ
μ€λμ Kotlin νλ‘νΌν° μμμ λν΄
곡λΆν λ΄μ©μ μ 리ν΄λ³΄λ € ν©λλ€.
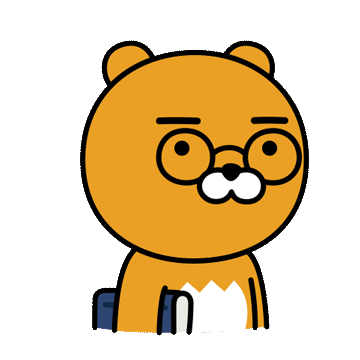
νλ‘νΌν° μμμ΄λ?
νλ‘νΌν°μ κ° μ€μ (set)/κ°μ Έμ€κΈ°(get)λ₯Ό λ€λ₯Έ class λ° λ©μλλ₯Ό ν΅ν΄ μμνλλ‘ νλ λμμΌλ‘
λ·λ°μΉ¨ νλ(Backing field)μ λ§μ°¬κ°μ§λ‘
- val μ κ²½μ° : getValue()λ§ κ΅¬ν
- var μ κ²½μ° : setValue() / getValue() λλ€ κ΅¬ν
by ν€μλλ₯Ό μ΄μ©νμ¬ μμν κ°μ²΄λ₯Ό νλ‘νΌν° λ€μ λͺ μν©λλ€.
μμΈλ¬ μμν getValue()μ setValue()λ operator ν€μλλ₯Ό μ¬μ©νμ¬ κ΅¬νν©λλ€.
μμ μ½λλ μλμ κ°μ΅λλ€.
import kotlin.reflect.KProperty
data class Customer(val id: Int = -1, val name: String = "", var address: String = "", var phone: String = "")
val dataBase = mutableSetOf(
Customer(1, "μ μ 1", "μμΈμ κΈμ²κ΅¬", "010-3745-2833"),
Customer(2, "μ μ 2", "μμΈμ λ
Έμꡬ", "010-3745-2323"),
Customer(3, "μ μ 3", "μμΈμ κΈμ²κ΅¬", "010-3745-3278"),
Customer(4, "μ μ 4", "κ²½κΈ°λ νμ΄μ", "010-3745-2454")
)
/* getValue μ λμνλ ν¨μ */
fun queryForCustomer(id: Int) = dataBase.find { customer ->
customer.id == id
} ?: Customer(-1, "μλ£μμ", "μλ£μμ", "μλ£μμ")
/* setValue μ λμνλ ν¨μ */
fun updateForCustomer(customer: Customer) = dataBase.add(customer)
/*
id λ₯Ό μ΄μ©νμ¬ DB(μ¬κΈ°μ κ°λ¨ν λ°°μ΄)μμ Customer κ°μ²΄λ₯Ό
getter/setter λ₯Ό μμ(PersonDelegate)νμ¬ μ²λ¦¬
*/
class PersonKT {
var id : Int = -1
var customerInfo: Customer by PersonDelegate()
}
class PersonDelegate{
operator fun getValue(personKT: PersonKT, property: KProperty<*>): Customer {
return queryForCustomer(personKT.id)
}
operator fun setValue(personKT: PersonKT, property: KProperty<*>, value: Customer) {
if(updateForCustomer(value)){
println("μ μ μ
λ ₯!")
}else{
println("μ
λ ₯ μ€ν¨!")
}
}
}
fun main() {
val personKT = PersonKT()
personKT.customerInfo = Customer(5, "μ μ 5", "μμΈμ κ°λ¨κ΅¬", "010-3214-5678")
personKT.id = 5 //setter νΈμΆν λ μμμ΄ μΌμ΄λ μ
λ ₯
println(personKT.customerInfo) //getter νΈμΆ μ μμνμ¬ μ€ν
}
μΆκ°μ μΌλ‘ kotlin.property.observableμ ν΅ν μμλ κ°λ₯νλ°
ν΄λΉ ν¨μλ κ°μ΄ λ³κ²½λλ κ²μ κ΄μ°°νμ¬ λμμ μνν©λλ€.
μ½λλ₯Ό 보며 λ€μ μ€λͺ λλ¦¬κ² μ΅λλ€.
var monthOfSalary : Long by Delegates.observable(1L)
{ _ , oldValue , newValue ->
val mSalary = salary/12 - salary/12* TAX_RATE
val previousSalary = when(oldValue){
3L , 6L , 9L , 12L -> (mSalary + mSalary*0.5).toLong()
else -> mSalary.toLong()
}
val currentSalary = when (newValue) {
3L, 6L, 9L, 12L -> {
(mSalary + mSalary*0.5).toLong()
}
else -> mSalary.toLong()
}
println("${oldValue}μμ κΈμ¬λ ${NumberFormat.getCurrencyInstance().format(previousSalary)}μ΄κ³ ${newValue}μμ κΈμ¬λ $" +
"${NumberFormat.getCurrencyInstance().format(currentSalary)}μ
λλ€.")
}
μμ κ°μ΄ Delgate.observableμ λλ€λ₯Ό ν΅ν΄ ꡬνν©λλ€.
νλΌλ―Έν°λ μ΄κΈ°κ°μ λνλ΄λ©°
λλ€ ν¨μ ꡬνλΆμμ μ λ¬ν΄μ£Όλ μΈμκ°μ κ°κ° Kproperty<*> , oldValue(μ΄μ κ°) , newValue(λ³κ²½λ κ°)μΌλ‘
μ¬μ©νμ§ μμ κ°μ ( _ ) λ‘ μλ΅μ΄ κ°λ₯ν©λλ€.
μ μ½λλ 3,6,9,12 μμ 보λμ€λ₯Ό λ°λ λ€λ κ°μ νμ μκΈ (monthOfSalary)μ Delgate.observableμ μμ
νμ¬ κ³μ°νλλ‘ νλ λΆλΆμ λλ€. π€
μ μ½λλ₯Ό μμ©νμ¬ 1~12μ μ 체 μκΈμ μΆλ ₯νλ νλ‘κ·Έλ¨μ λ§λ€μ΄ 보μμ΅λλ€.
import java.text.NumberFormat
import java.time.format.FormatStyle
import java.util.*
import kotlin.math.abs
import kotlin.properties.Delegates
class EmployeeKT(val empName : String , private val salary : Long = 0L , val department : String = "κ°λ°λΆ" , var empNumber : String = "") {
var monthOfSalary : Long by Delegates.observable(1L)// νλΌλ―Έν°λ μ΄κΈ°κ°
{ _ , oldValue , newValue ->
val mSalary = salary/12 - salary/12* TAX_RATE
val previousSalary = when(oldValue){ // μ΄μ λ¬ μκΈ
3L , 6L , 9L , 12L -> (mSalary + mSalary*0.5).toLong()
else -> mSalary.toLong()
}
val currentSalary = when (newValue) { // μ΄λ²λ¬ μκΈ
3L, 6L, 9L, 12L -> {
(mSalary + mSalary*0.5).toLong()
}
else -> mSalary.toLong()
}
println("${oldValue}μμ κΈμ¬λ ${NumberFormat.getCurrencyInstance().format(previousSalary)}μ΄κ³ ${newValue}μμ κΈμ¬λ $" +
"${NumberFormat.getCurrencyInstance().format(currentSalary)}μ
λλ€.")
}
init {
empNumber = abs(Random().nextInt()).toString()
}
}
fun main() {
val emp = EmployeeKT("Lee Yo han" , 120_000_000)
for(i in 2..12) {
emp.monthOfSalary = i.toLong()
}
}
κ²°κ³Όκ°μ μλμ κ°μ΅λλ€. π